Namespace
Organize resources in a namespace
Virtual cluster inside a cluster
Default namespaces
- kube-system, system processes
- kube-public, publicely accessible data
- kube-node-lease, heartbeats of nodes
- default, default namespace
Create Namespace
# create a namespace
# kubectl create namespace [namespaceName]
kubectl create namespace count-ns
# create a resource in a namespace
kubectl apply -f redis-deployment.yaml --namespace=count-ns
# namespace.yaml
apiVersion: v1
kind: Namespace
metadata:
name: count-ns
List Resource
# list namespace
kubectl get namespaces
# list resources in a namespace
# kubectl -n [namespaceName] get [resource]
kubectl -n count-ns get all
kubectl -n count-ns get services
kubens
# install kubens
brew install kubectx
# list namespace and highlight the current namespace
# by default, "default" is the current namespace
kubens
# switch namespace
# kubens [namespaceName]
kubens count-ns
kubenctl get all # list resources in current namespace
Deployment
Cannot access most resource from another namespace
Each namespace must define own ConfigMap, Secret
Service can be shared, access a service in a different namespace with [serviceName].[namespaceName]
Some components cannot be created in namespace, such as volume, node
1. Create a namespace for each team or project
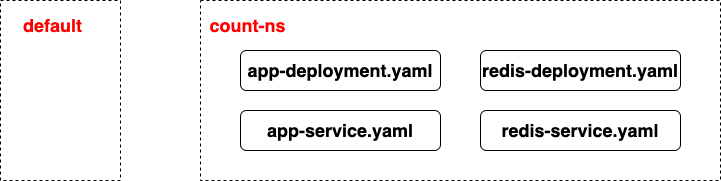
# app.yaml
apiVersion: v1
kind: Namespace
metadata:
name: count-ns
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: webapp-deployment
labels:
app: webapp
namespace: count-ns
spec:
replicas: 1
selector:
matchLabels:
app: webapp # let deployment know which pod for this deployment
template: # configuration for pods
metadata:
labels:
app: webapp # each pod has a unique name, but pods can share the same label
spec:
containers: # containers in a pod, usually add one container per pod
- name: webapp
image: lchenlangley/count # pull image from local
imagePullPolicy: Never
ports:
- containerPort: 5000
env:
- name: MYREDIS_HOST
value: redis-service
---
apiVersion: v1
kind: Service
metadata:
name: webapp-service # service name accessed by app
namespace: count-ns
spec:
selector:
app: webapp # match the pod labels
ports:
- protocol: TCP
port: 5000 # service port
targetPort: 5000 # pod port
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-deployment
labels:
app: redis
namespace: count-ns
spec:
replicas: 1
selector:
matchLabels:
app: redis # let deployment know which pod for this deployment
template: # configuration for pods
metadata:
labels:
app: redis # each pod has a unique name, but pods can share the same label
spec:
containers: # containers in a pod, usually add one container per pod
- name: redisdb
image: redis:alpine # pull image from Docker Hub
ports:
- containerPort: 6379
---
apiVersion: v1
kind: Service
metadata:
name: redis-service # service name accessed by app
namespace: count-ns
spec:
selector:
app: redis # match the pod labels
ports:
- protocol: TCP
port: 6379 # service port
targetPort: 6379 # pod port
# deploy resources
kubectl apply -f app.yaml
# access app
kubectl -n count-ns port-forward svc/webapp-service 8000:5000
http://localhost:8000/
# remove resources
kubectl delete -f app.yaml
2. Resources grouped in namespaces
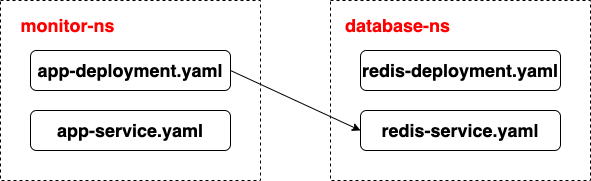
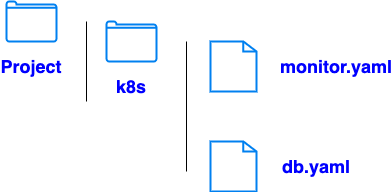
# monitor.yaml
apiVersion: v1
kind: Namespace
metadata:
name: monitor-ns
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: webapp-deployment
labels:
app: webapp
namespace: monitor-ns
spec:
replicas: 1
selector:
matchLabels:
app: webapp # let deployment know which pod for this deployment
template: # configuration for pods
metadata:
labels:
app: webapp # each pod has a unique name, but pods can share the same label
spec:
containers: # containers in a pod, usually add one container per pod
- name: webapp
image: lchenlangley/count # pull image from local
imagePullPolicy: Never
ports:
- containerPort: 5000
env:
- name: MYREDIS_HOST
value: redis-service.db-ns
---
apiVersion: v1
kind: Service
metadata:
name: webapp-service # service name accessed by app
namespace: monitor-ns
spec:
selector:
app: webapp # match the pod labels
ports:
- protocol: TCP
port: 5000 # service port
targetPort: 5000 # pod port
# db.yaml
apiVersion: v1
kind: Namespace
metadata:
name: db-ns
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-deployment
labels:
app: redis
namespace: db-ns
spec:
replicas: 1
selector:
matchLabels:
app: redis # let deployment know which pod for this deployment
template: # configuration for pods
metadata:
labels:
app: redis # each pod has a unique name, but pods can share the same label
spec:
containers: # containers in a pod, usually add one container per pod
- name: redisdb
image: redis:alpine # pull image from Docker Hub
ports:
- containerPort: 6379
---
apiVersion: v1
kind: Service
metadata:
name: redis-service # service name accessed by app
namespace: db-ns
spec:
selector:
app: redis # match the pod labels
ports:
- protocol: TCP
port: 6379 # service port
targetPort: 6379 # pod port
# deploy
kubectl create -f k8s
# access service
kubectl -n monitor-ns port-forward svc/webapp-service 8000:5000
http://localhost:8000/
# delete
kubectl delete -f k8s
3. Resources sharing
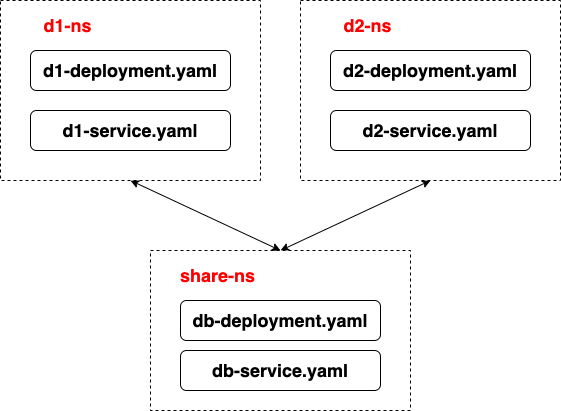
Delete Namespace
Deleting the namespace also deletes all the residing components
Removing all deployments within a namespace does not remove the namespace
# kubectl delete namespace [namespaceName]
kubectl delete namespace count-ns
Reference